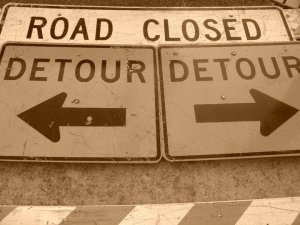
Recently I had to customize a form for a client using Gravity Forms. The basic concept was creating a single form with a drop-down to select the user the form would email. I figured there would be a plugin or built-in option for this, but alas I was disappointed. So let’s build it!
There is a “Configure Routing” option in the notifications and for a simple form you can add various conditional elements. For example, you can say if “Josh” is selected in the “Email to” field, send to a specific email address. However, this has to be manually entered, both the conditional statement and the send-to email address. I needed the ability to auto-populate the drop-down with WordPress users and send the notification (in my case a contact form message) to that selected user. Luckily, there’s a filter that we can use.
Populating the Drop-down with Users
First, be sure you have a drop-down field created on your form. An important step is creating a custom CSS class for the drop-down field, that way we can find the correct drop-down to populate (in the “Advanced” tab of the field options). For this example I’ve given the field class the identifier “user-emails.”
Next, add this to your functions.php
file (or, if you’re being really future-proof place it in a plugin file).
In this example our form ID is 1, but change it in the filter name to match your form ID. Also, change the “user-emails” CSS class to whatever class your drop-down field is using.
What this code does:
- It takes the
$form
arrayย and goes through each field in the foreach statement. - Then we place a if statement to make sure we find the field we are looking for – a select field with a specific CSS class.
- After that, we build a new array of
$choices
, each with a ‘text’ and ‘value’ for the field. In this case, we’re using user data, so… - Create a new
WP_User_Query
to find all the users. We’re only using the user id, display name, and the user email. - Add the user display name for the text and the user id for the value.
- Set the field’sย choices with our new array and return the
$form
.
Some things to note:
- Why not use the email address for the value and pass that into the notification function (below)? Well, part of the reason I created this in the first place was to hide email addresses on the front end. Plus, it is easier to use the user id when utilizing the populate dynamically feature.
- You can use other methods to filter the users in the initial query. If you are looking to only have, say, the contributors or admins. I’d recommend using the wonderful GenerateWP tool. If you do this, you can likely remove theย
&& user_can( $user->id, 'edit_posts' )
conditional.
Using that Email for the Notification Send To Field
The next step is to use that field’s value (user id) and generate the notification’s send to email address. This gets added to your functions.php
(or plugin) file:
Again, change the 1 in the filter name to your form ID and the CSS class like above.
What this code does:
- We’re filtering the notification here, and we’ve been given the
$notification
,$form
and$entry
arrays. The first two should be straight forward by their names, the$entry
is the submitted values of your form. - We’ll loop through the form’s fields and find the one we want (similar to the previous function).
- Once we find the right field, we grab the field id and use the
$entry
array to get the value selected (in this case, the user id). - Using that user id and
get_the_author_meta
we’ll find the user_email we need. - Set the notification ‘to’ field and return the notification.
That’s pretty straight-forward and basically it.
Wrap it up and Resources
You can view the entire gist here. Some valuable resources for this little project:
- Gravity Form Documentation:
- GenerateWP
- WP_User_Query Codex
- Gravity Forms Notifications: route to an email that is stored in a custom field
- Populating Gravity Form Drop-Down (pastie)
- WordPress Codex – get_the_author_meta
Hope that was helpful! It is pretty slick once it’s working.
Leave a Reply